API Documentation
for Web3
Discover our comprehensive API documentation for QuickNode developer tools and 20+ supported blockchains. Learn how to call hundreds of different RPC methods across various chains and APIs including Core API, Token API, NFT API, and more using JavaScript, Python, Ruby, and popular Web3 SDKs.
Get Started with RPC/REST API1import { Core } from '@quicknode/sdk'23const core = new Core({4endpointUrl: 'https://{your-endpoint-name}.quiknode.pro/{your-token}/',5})67core.client.getBlockNumber()8
Core API
Use the quickest and most reliable blockchain API with unparalleled latency, reliability, and scalability across all major blockchains.
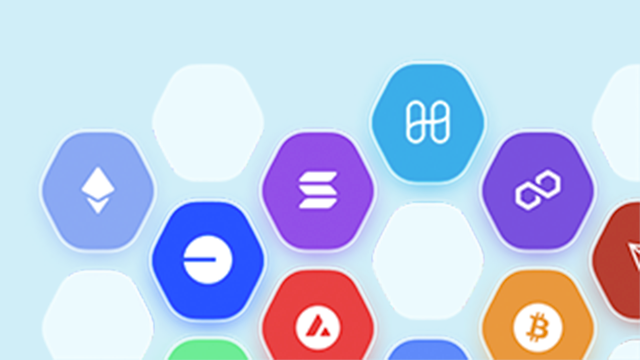
QuickAlerts
Leverage a custom real-time blockchain data feed, advanced alerting, and include real-time blockchain event driven logic into your workflows.
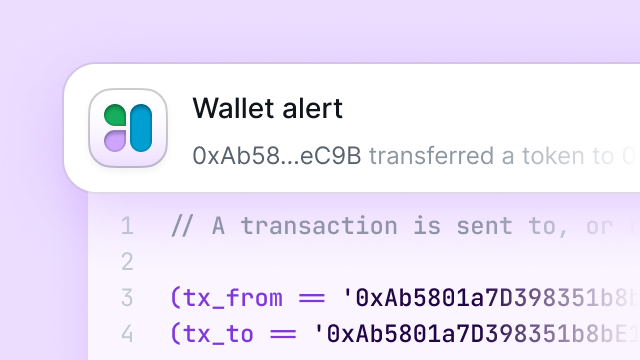
NFT API
Retrieving NFT metadata from the blockchain is tedious and time-consuming. Our NFT API does the heavy lifting for you across Solana and Ethereum NFTs.
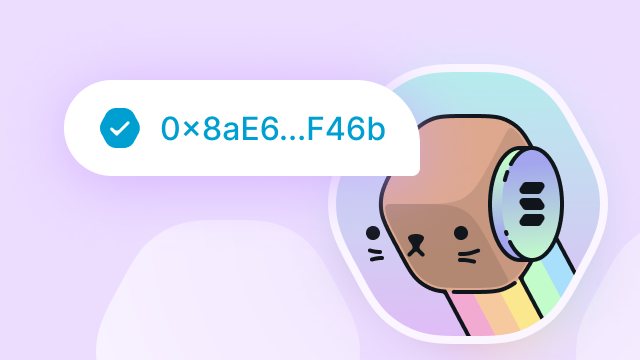
Token API
Our Token API lets you easily look up ERC-20 tokens by wallet, token transfers, and token details instantly. No indexing required!
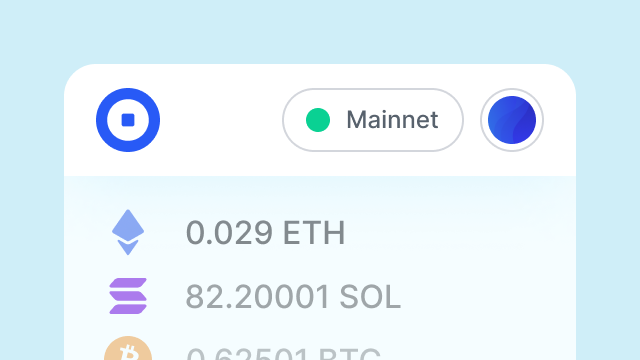